REST stands for Representational State Transfer, It's a kind of Application Programming Interface that provides access to system resources through HTTP protocol. REST API uses the following methods to access data from REST Server.
GET: Used to read the data from the server.
POST: Used to create data on a server.
PATCH: Used to update the data on the server.
DELETE: Used to delete the data from the server.
Drupal core includes RESTful Web Services that come with a few predefined REST API routes, we can utilize them to export, update and create content. Drupal REST module depends on serialization service module responsible for serializing data into formats like JSON and XML.
Drupal Modules
RESTful Web Services - Provide access to entities and other resources through predefined REST endpoints, Define resource plugin type to extend and build our own REST endpoint. also provides ViewsDisplay
, ViewRows
, and ViewsStyle
to develop a REST endpoint through View.
HTTP Basic Authentication - Provide HTTP basic authentication provider to access secure resources.
Serialization - Required by RESTful Web Services module, This module registers serialization service and further provides services for normalization and denormalization of entities.
REST UI - It's a contributed module that provides a user interface to manage REST resources.
Getting Started
Start by downloading rest_ui
module through composer, Then enable modules either by Drush or by admin dashboard.
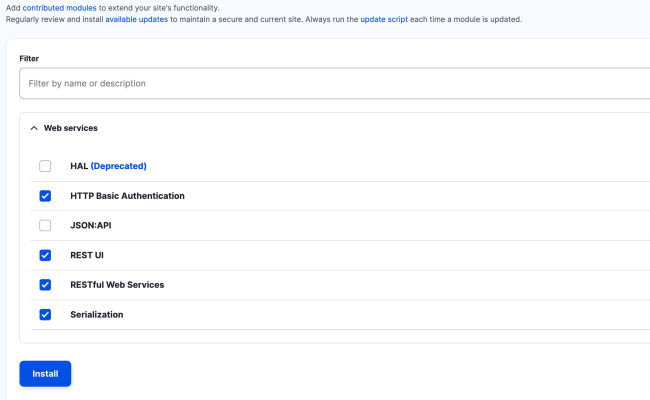
Enable REST Resource
Open the REST Resource admin page (Configuration > Web Services > REST). This page will present a list of predefined resources, Enable REST Resource for content.
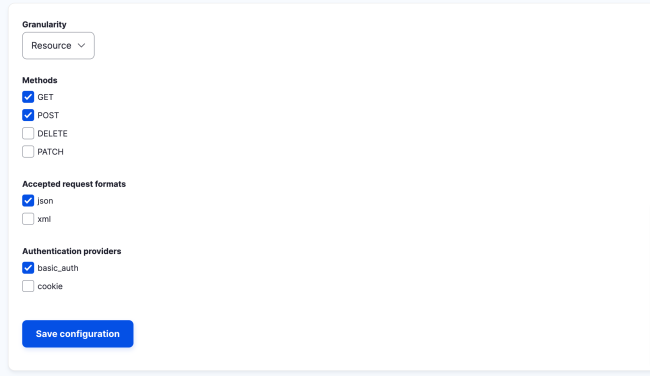
Now, we can use postman or some other HTTP Client to request and test the REST API.
Get node content using REST API
No authentication is applicable on this, We have to provide resource URL and Content-Type headers and we will get resource data.
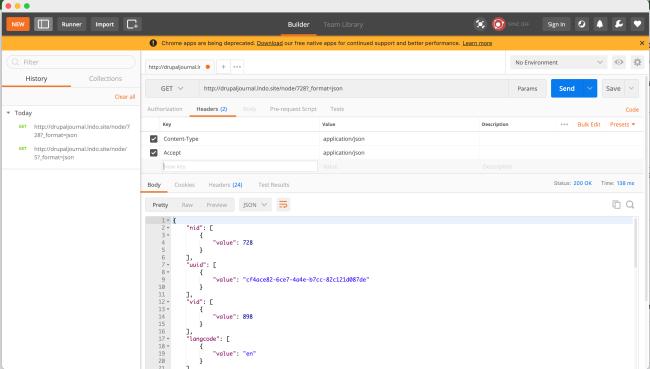
Create a new node using REST API
Basic auth is provided for authentication with X-CSFF-Token, and the POST method is used to post JSON data of node.

Get X-CSRF-Token
<?php
// Exported from postman.
$request = new HttpRequest();
$request->setUrl('https://example.com/session/token');
$request->setMethod(HTTP_METH_GET);
$request->setHeaders(array(
'cache-control' => 'no-cache'
));
try {
$response = $request->send();
echo $response->getBody();
} catch (HttpException $ex) {
echo $ex;
}
POST node data
<?php
// Exported form postman.
$request = new HttpRequest();
$request->setUrl('http://example.com/node');
$request->setMethod(HTTP_METH_POST);
$request->setQueryData(array(
'_format' => 'json'
));
$request->setHeaders(array(
'cache-control' => 'no-cache',
'x-csrf-token' => '{your_token}', // See above example.
'authorization' => 'Basic {basic_auth_token}', // Generated by postman after providing username and password to authorization tab.
'accept' => 'application/json',
'content-type' => 'application/json'
));
$request->setBody('{
"type": [
{
"target_id": "article"
}
],
"title": [
{
"value": "Test content 22"
}
]
}');
try {
$response = $request->send();
echo $response->getBody();
} catch (HttpException $ex) {
echo $ex;
}
Create a view with REST export
Create a view, and enable REST Export from the same screen.
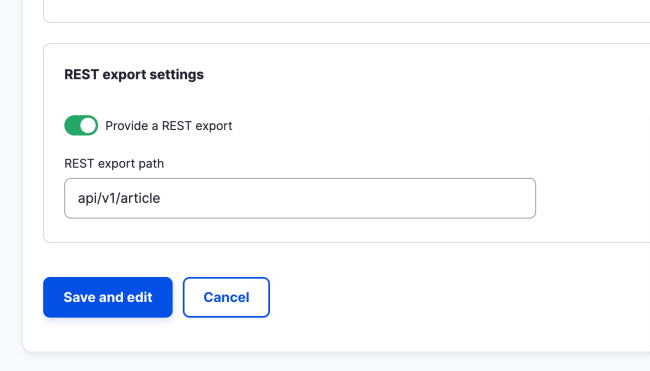
Create a custom resource endpoint
RESTful Web Services is an extensible module, It provides a Resource plugin type, and we can create several other resources by creating plugins.
You can check a detailed article about how to create GET and POST resources here https://drupaljournal.com/article/drupal/create-custom-rest-resource-get-and-post-drupal